Competitive Programming in Python: 128 Algorithms to Develop Your Coding Skills Free PDF Learn to write DAX: a practical guide to learning Power Pivot for Excel and Power BI Python Machine Learning: The Complete Guide to Understand Python Machine Learning for Beginners and Artificial Intelligence. This chapter will get you up and running with Python, from downloading it to writing simple programs. 1.1 Installing Python Go towww.python.organd download the latest version of Python (version 3.5 as of this writing). It should be painless to install. If you have a Mac or Linux, you may already have Python on your. Python 3 Cheat Sheet This is the best single cheat sheet. It uses every inch of the page to deliver value and covers everything you need to know to go from beginner to intermediate. Topics covered include container types, conversions, modules, math, conditionals, and formatting to name a few. The functionalities discussed have very speci c applications and act like a SHORTCUT or a CHEAT-SHEET in competitive coding. Competitive Programming Python 7.
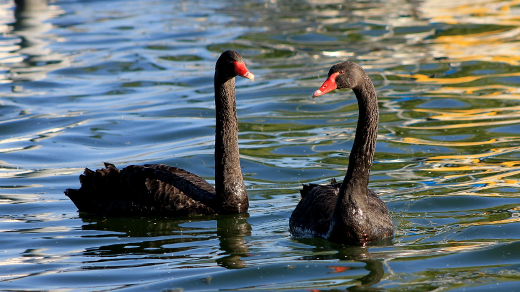
- Related Questions & Answers
- Selected Reading
Python is one of the preferred languages among coders for most of the competitive programming challenges. Most of the problems are easily computed in a reasonable time frame using python.
For some of the complex problem, writing fast-enough python code is often a challenge. Below are some of the pythonic code constructs that help to improve the performance of your code in competitive coding −
1. Strings concatenation: Do not use the below construct.
Above method gives huge time overhead.Instead, try to use this (join method) −
2. The Map function
Generally, you have an input in competitive coding, something like −
1234567
To get them as a list of numbers simply
Always use the input() function irrespective of the type of input and then convert it using the map function.
The map function is one of the beautiful in-built function of python, which comes handy many times. Worth knowing.
3. Collections module
In case we want to remove duplicates from a list. While in other languages like Java you may have to use HashMap or any other freaky way, however, in pytho it's simply

Also, be careful to use extend() and append() in lists, while merging two or more lists.
4. Language constructs
It's better to write your code within functions, although the procedural code is supported in Python.
is much better than
It is faster to store local variables than globals because of the underlying Cpython implementation.
5. Use the standard library:
It’s better to use built-in functions and standard library package as much as possible. There, instead of −
Use this −
Likewise, try to use the itertools(standard library), as they are much faster for a common task. For example, you can have something like permutation for a loop with a few lines of code.
6. Generators
Generators are excellent constructs to reduce both, the memory footprint and the average time complexity of the code you’ve written.
Basics
Print a number | print(123) |
Print a string | print('test') |
Adding numbers | print(1+2) |
Variable assignment | number = 123 |
Print a variable | print(number) |
Function call | x = min(1, 2) |
Comment | # a comment |
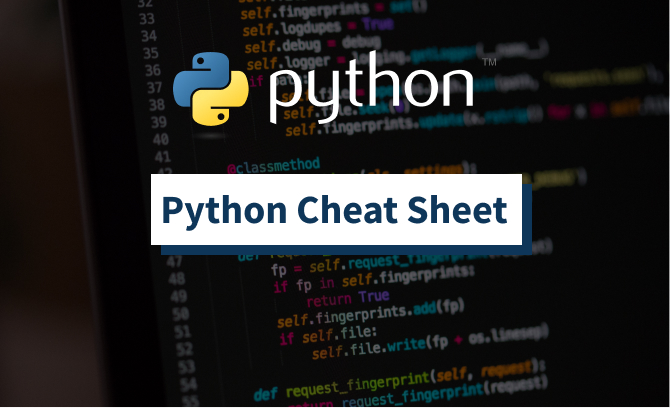
Types
Integer | 42 |
String | 'a string' |
List | [1, 2, 3] |
Tuple | (1, 2, 3) |
Boolean | True |
Useful functions
Write to the screen | print('hi') |
Calculate length | len('test') |
Minimum of numbers | min(1, 2) |
Maximum of numbers | max(1, 2) |
Cast to integer | int('123') |
Cast to string | str(123) |
Cast to boolean | bool(1) |
Range of numbers | range(5, 10) |
Other syntax
Return a value | return 123 |
Indexing | 'test'[0] |
Slicing | 'test'[1:3] |
Continue to next loop iteration | continue |
Exit the loop | break |
List append | numbers = numbers + [4] |
List append (with method call) | numbers.append(4) |
List item extraction | value = numbers[0] |
List item assignment | numbers[0] = 123 |
Python Cheat Sheet Download
Terminology
syntax | the arrangement of letters and symbols in code |
program | a series of instructions for the computer |
write text to the screen | |
string | a sequence of letters surrounded by quotes |
variable | a storage space for values |
value | examples: a string, an integer, a boolean |
assignment | using = to put a value into a variable |
function | a machine you put values into and values come out |
call (a function) | to run the code of the function |
argument | the input to a function call |
parameter | the input to a function definition |
return value | the value that is sent out of a function |
conditional | an instruction that's only run if a condition holds |
loop | a way to repeatedly run instructions |
list | a type of value that holds other values |
tuple | like a list, but cannot be changed |
indexing | extracting one element at a certain position |
slicing | extracting some elements in a row |
dictionary | a mapping from keys to values |
Reminders
- Strings and lists are indexed starting at 0, not 1
- Print and return are not the same concept
- The return keyword is only valid inside functions
- Strings must be surrounded by quotes
- You cannot put spaces in variable or function names
- You cannot add strings and integers without casting
- Consistent indentation matters
- Use a colon when writing conditionals, function definitions, and loops
- Descriptive variable names help you understand your code better
Conditionals
Python Competitive Programming Cheat Sheet Pdf
Lists
Defining functions
Loops
Dictionaries
Comparisons
Equals | |
Not equals | != |
Less than | < |
Less than or equal | <= |
Greater than | > |
Useful methods
String to lowercase | 'xx'.lower() |
String to uppercase | 'xx'.upper() |
Split string by spaces | 'a b c'.split(' ') |
Remove whitespace around string | ' a string '.strip() |
Combine strings into one string | ' '.join(['a', 'b']) |
String starts with | 'xx'.startswith('x') |
String ends with | 'xx'.endswith('x') |
List count | [1, 2].count(2) |
List remove | [1, 2].remove(2) |
Dictionary keys | {1: 2}.keys() |
Dictionary values | {1: 2}.values() |
Dictionary key/value pairs | {1: 2}.items() |
Other neat bonus stuff
Python Competitive Programming Cheat Sheet Free

Zip lists | zip([1, 2], ['one', 'two']) |
Set | my_set = {1, 2, 3} |
Set intersection | {1, 2} & {2, 3} |
Set union | {1, 2} | {2, 3} |
Index of list element | [1, 2, 3].index(2) |
Sort a list | numbers.sort() |
Reverse a list | numbers.reverse() |
Sum of list | sum([1, 2, 3]) |
Numbering of list elements | for i, item in enumerate(items): |
Read a file line by line | for line in open('file.txt'): |
Read file contents | contents = open('file.txt').read() |
Random number between 1 and 10 | import random; x = random.randint(1, 10) |
List comprehensions | [x+1 for x in numbers] |
Check if any condition holds | any([True, False]) |
Check if all conditions hold | all([True, False]) |
Cheat Sheets For Python
Missing something?
Let us know here.